Dictionary class extensions (CopyTo, Sort) (C#)
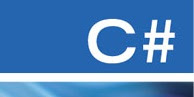
It seems that are not any CopyTo and Sort functions in the Dictionary class that resides in the Systems.Collection.Generic namespace. The OrderBy<> extension method used by Linq is not very practical to me, because it does not return a dictionary object. Here is my extension method CopyTo, applicable to generic Dictionary objects, allowing to define a part of the dictionary:
public static void CopyTo<T, V>(this Dictionary<T, V> source, Dictionary<T, V> target)
{
if (target == null) target = new Dictionary<T, V>();
foreach (KeyValuePair<T, V> entry in source)
target.Add(entry.Key, entry.Value);
}
public static void CopyTo<T, V>(this Dictionary<T, V> source, Dictionary<T, V> target, int start)
{
if (target == null) target = new Dictionary<T, V>();
int iEntry = 0;
foreach (KeyValuePair<T, V> entry in source)
{
if (iEntry++ >= start) target.Add(entry.Key, entry.Value);
}
}
public static void CopyTo<T, V>(this Dictionary<T, V> source, Dictionary<T, V> target, int start, int end)
{
if (target == null) target = new Dictionary<T, V>();
int iEntry = 0;
foreach (KeyValuePair<T, V> entry in source)
{
if (iEntry >= start && iEntry <= end) target.Add(entry.Key, entry.Value);
iEntry++;
}
}
There is a SortedDictionary object that allows to sort dictionary entries, but only by Key. I have created a convenient Sort method that allows to sort in a custom way, just like the List object. Note that this is not very practical for large arrays, because a copy of the key-value entries is created. There are many custom Sort functions that we can define to improve performance.
public static void Sort<T, V>(this Dictionary<T, V> source, Comparison<KeyValuePair<T, V>> comparison)
{
//loads the entries to a list
List<KeyValuePair<T, V>> entries = new List<KeyValuePair<T, V>>(source);
//sort them according to the comparison function
entries.Sort(comparison);
//clear all unsorted entries
source.Clear();
//read the sorted entries
foreach (var entry in entries) source.Add(entry.Key, entry.Value);
}
public static void Sort<T, V>(this Dictionary<T, V> source, IComparer<KeyValuePair<T, V>> comparer)
{
//loads the entries to a list
List<KeyValuePair<T, V>> entries = new List<KeyValuePair<T, V>>(source);
//sort them according to the comparison function
entries.Sort(comparer);
//clear all unsorted entries
source.Clear();
//readd the sorted entries
foreach (var entry in entries) source.Add(entry.Key, entry.Value);
}
Note that all extension methods should be defined inside static classes.
Comments