Array Slice in C#
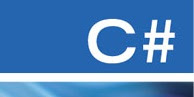
Unfortunately the array objects in C# do not have functions to return parts of them and I think it is not efficient to use Linq extension functions (in these cases). That is the reason why I have created a convenient Slice extension function:
public static class ArrayExtensions
{
//returns a part of the source array
public static T[] Slice<T>(this T[] source, int start, int end)
{
int count = end - start + 1;
T[] target = null;
if (count > 0)
{
target = new T[count];
for (int i = start; i <= end; i++)
target[i - start] = source[i];
}
return target;
}
}
Here is an example, that shows to you the usage of the generic Slice function:
string[] linesSrc, lineTo;
...
//return a sub-array of the first three elements of the linesSrc array
lineTo = linesSrc.Slice<string>(0, 2);
That's the good part of the extension functions: it is general and can be used for all type of arrays.
Comments