Cross thread UI calls in C#
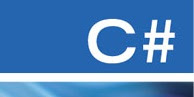
If you try to call UI functions through threads other than the one the created the UI elements then an error will occur. To avoid those type of errors we need to have thread-safe calls to the corresponding Windows controls. For example, to make a thread-safe call for a textbox control, the following is valid:
private void SetText(string text)
{
if (txtCommand.InvokeRequired)
this.Invoke(new Action<string>(SetText), text);
else
txtCommand.Text = text;
}
The Invoke function will be called if the function is called from a thread other than the one that created the control. Here is a second example that will take two arguments; the first one is the control itself.
private void EnableButton(Button button, bool enabled)
{
// InvokeRequired compares the thread ID of the
// calling thread to the thread ID of the creating thread.
// If these threads are different, it returns true.
if (button.InvokeRequired)
button.Invoke(new Action<Button, bool>(EnableButton),
new object[] { button, enabled });
else
button.Enabled = enabled;
}
After some similar functions that I wrote, I noticed that most of the functionality was similar and that's why I wrote a more generic function for the job:
public void SafeSetValue<ControlType, PropertyType>(ControlType control, string controlProperty, PropertyType value) where ControlType : Control
{
if (control.InvokeRequired)
control.Invoke(new Action<ControlType, string, PropertyType>(SafeSetValue<ControlType, PropertyType>),
control, controlProperty, value);
else
{
System.Reflection.PropertyInfo p = typeof(ControlType).GetProperty(controlProperty);
p.SetValue(control, value, null);
}
}
And here are two equivalent ways to enable a button control using the functions defined above (one of the following is needed to be called):
//1: calling the 1st function
EnableButton(button, true);
//2: calling the generic function
SafeSetValue<Button, bool>(button, "Enabled", true);
Comments