Automate Excel using C#
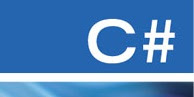
Excel can be easily automated using C#. At first you must check that the Microsoft.Office.Interop.Excel is referenced. When the Framework 4.0 is targeted you should check that the Microsoft.CSharp.dll is referenced too.
using System; using Excel = Microsoft.Office.Interop.Excel; //... Excel.Application xlApp = new Excel.Application(); //_Application xlApp = new Excel.Application(); xlApp.Visible = true; //create a workbook Workbook wb = xlApp.Workbooks.Add(XlWBATemplate.xlWBATWorksheet); //or open a workbook Workbook wb = xlApp.Workbooks.Open("c:\\book.xlsx"); //get the first worksheet Worksheet ws = (Worksheet)wb.Worksheets[1]; //Worksheets["Sheet1"] //create a worksheet Worksheet ws2 = (Worksheet)wb.Worksheets.Add(); //retrieve a range Range range = (Range)ws.Range["a1"]; Range range2 = (Range)ws.Cells[1, 1]; //the A1 cell range.Value="arkoudaki"; //alternative way to do the same thing //range2.set_Value(XlRangeValueDataType.xlRangeValueDefault,"arkouda");
Comments